Introduction
Verify Account UI is a web-based project. This dialogue message appears when you verify your email. It is coded in HTML5, CSS, and JavaScript.
In this tutorial, we will be creating a simple verify account interface. This message appears during registering on a website. First, we need to create a folder with html, css, and js files. You need to enter the six-digit code which you receive in the email. When you enter those codes your email will be verified. This verify account project has a simple layout.
This verify account project’s functionality is made with CSS and JavaScript. HTML is basically for adding text and numbers on screen. When you enter a number you will see a pop up shadow box effect on it. We are using google fonts in this project. You need to run index.html file in the browser to run the project. See the screenshot below.
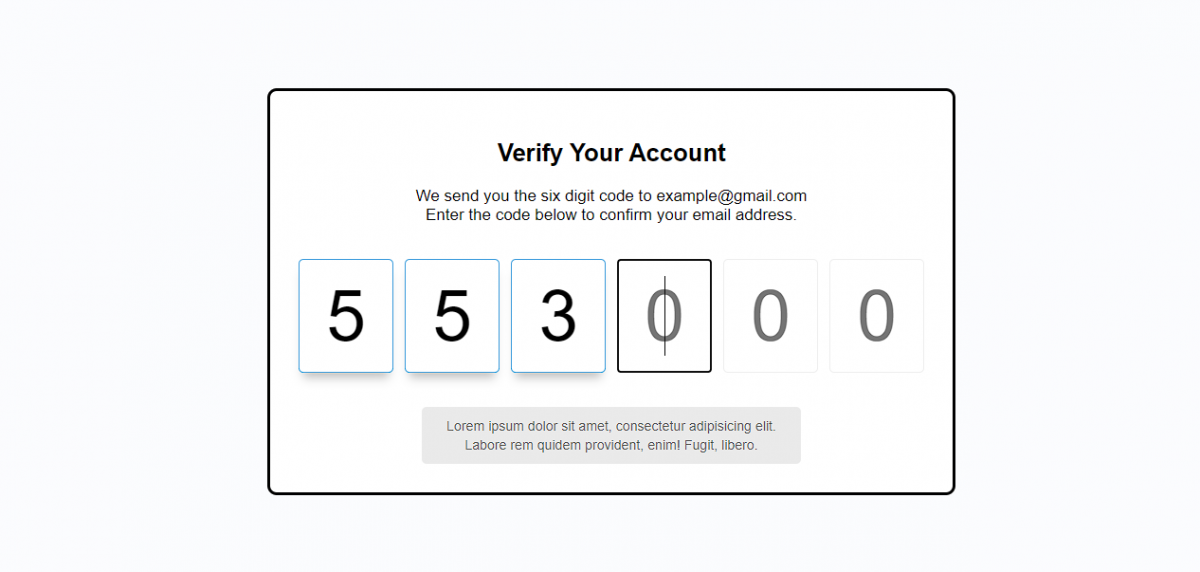
Verify account html, css & js codes
HTML will be used for displaying text and number placeholders on the screen. Make sure you have an internet connection. We are using google font cdn.
index.html
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <link rel="stylesheet" href="style.css" /> <title>Verify Account</title> </head> <body> <div class="container"> <h2>Verify Your Account</h2> <p>We send you the six digit code to example@gmail.com <br/> Enter the code below to confirm your email address.</p> <div class="code-container"> <input type="number" class="code" placeholder="0" min="0" max="9" required> <input type="number" class="code" placeholder="0" min="0" max="9" required> <input type="number" class="code" placeholder="0" min="0" max="9" required> <input type="number" class="code" placeholder="0" min="0" max="9" required> <input type="number" class="code" placeholder="0" min="0" max="9" required> <input type="number" class="code" placeholder="0" min="0" max="9" required> </div> <small class="info"> Lorem ipsum dolor sit amet, consectetur adipisicing elit. Labore rem quidem provident, enim! Fugit, libero. </small> </div> <script src="script.js"></script> </body> </html>
We will be using CSS for styling the whole project. Such as background colour, icons, text, box layout, aligning, flex-items, colours and many more
style.css
@import url('https://fonts.googleapis.com/css?family=Roboto+Mono&display=swap'); * { box-sizing: border-box; } body { background-color: #fbfcfe; font-family: 'Roboto', sans-serif; display: flex; align-items: center; justify-content: center; height: 100vh; overflow: hidden; margin: 0; } .container { background-color: #fff; border: 3px #000 solid; border-radius: 10px; padding: 30px; max-width: 1000px; text-align: center; } .code-container { display: flex; align-items: center; justify-content: center; margin: 30px; } .code { border-radius: 5px; font-size: 75px; height: 120px; width: 100px; border: 1px solid #eee; margin: 1%; text-align: center; font-weight: 300; -moz-appearance: textfield; } .code::-webkit-outer-spin-button, .code::-webkit-inner-spin-button { -webkit-appearance: none; margin: 0; } .code:valid { border-color: #3498db; box-shadow: 0 10px 10px -5px rgba(0, 0, 0, 0.25); } .info { background-color: #eaeaea; display: inline-block; padding: 10px; line-height: 20px; max-width: 400px; color: #777; border-radius: 5px; } @media (max-width: 600px) { .code-container { flex-wrap: wrap; } .code { font-size: 60px; height: 80px; max-width: 70px; } }
The JavaScript code is used for making the shadow number box automatically. As you enter the numbers you will see the pop up shadow box immediately. Check the code below.
script.js
const codes = document.querySelectorAll('.code') codes[0].focus() codes.forEach((code, idx) => { code.addEventListener('keydown', (e) => { if(e.key >= 0 && e.key <=9) { codes[idx].value = '' setTimeout(() => codes[idx + 1].focus(), 10) } else if(e.key === 'Backspace') { setTimeout(() => codes[idx - 1].focus(), 10) } }) })
Although the code is provided in this tutorial. You can click the button below to get the full folder source code of this project.