Introduction
Double tap project is a web based project. It is coded in HTML5, CSS, and JavaScript. It displays heart when tapped on an image.
In this tutorial, we will be creating a simple double-tap heart button as in Instagram. This app will give you an idea of creating a double-tap and showing heart. First, we need to create a folder with HTML, CSS, and js files. This project’s functionality is made with CSS and JavaScript. HTML is basically used in this project for making id and class and adding text.
This project shows some text and images. When you double-tap the image a heart animation with appear and your likes will be counted. You will see an Instagram-like feature in this project. We are using google fonts in this project. You need to run the index.html file in the browser to run the project. See the screenshot below.
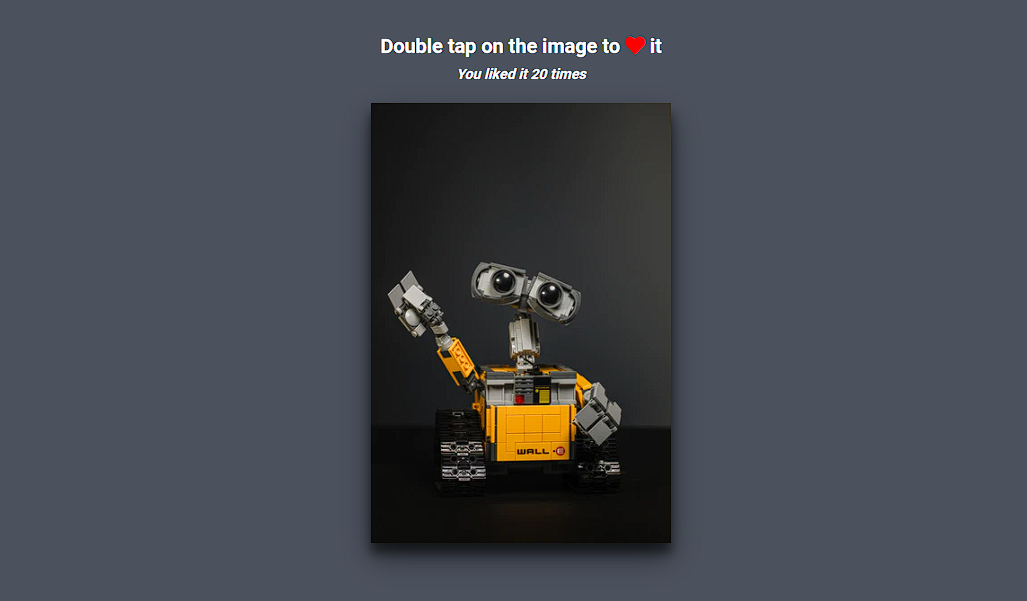
Double tap project source codes
HTML will be used for displaying text on the screen. Make sure you have an internet connection. We are using a random image from unsplash website.
index.html
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/5.15.2/css/all.min.css" integrity="sha512-HK5fgLBL+xu6dm/Ii3z4xhlSUyZgTT9tuc/hSrtw6uzJOvgRr2a9jyxxT1ely+B+xFAmJKVSTbpM/CuL7qxO8w==" crossorigin="anonymous" /> <link rel="stylesheet" href="style.css" /> <title>Double tap</title> </head> <body> <h3>Double tap on the image to <i class="fas fa-heart"></i> it</h3> <b><i><small>You liked it <span id="times">0</span> times</small></i></b> <div class="likeMe"> </div> <script src="script.js"></script> </body> </html>
We will be using CSS for styling the whole project. Such as background colour, icons, text, box layout, aligning, flex-items, colours and many more. Further we are adding animation in our project too.
style.css
@import url('https://fonts.googleapis.com/css2?family=Roboto:wght@400;700&display=swap'); * { box-sizing: border-box; margin: 0; padding: 0; } body { font-family: 'Roboto', sans-serif; background-color: #4b515d; text-align: center; overflow: hidden; margin: 0; } /* #37474f */ h3 { color: white; margin-top: 4%; text-align: center; } small { color: white; display: block; padding-top: 8px; margin-bottom: 20px; text-align: center; } .fa-heart { color: red; } .likeMe { height: 440px; width: 300px; background: url('https://images.unsplash.com/photo-1589254065909-b7086229d08c?ixid=MXwxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHw%3D&ixlib=rb-1.2.1&auto=format&fit=crop&w=334&q=80') no-repeat center center/cover; margin: auto; cursor: pointer; max-width: 100%; position:relative; box-shadow: 0 14px 28px rgba(0, 0, 0, 0.5), 0 10px 10px rgba(0, 0, 0, 0.5); } .likeMe .fa-heart { position: absolute; animation: grow 0.6s linear; transform: translate(-50%, -50%) scale(0); } @keyframes grow { to { transform: translate(-50%, -50%) scale(10); opacity: 0; } }
The JavaScript code is used for making the click counts. It also shows a heart animation when double click. As you double tap on image your click is recorded and a heart animation will be displayed. Check the code below.
script.js
const likeMe = document.querySelector('.likeMe') const times = document.querySelector('#times') let clickTime = 0 let timesClicked = 0 likeMe.addEventListener('click', (e) => { if(clickTime === 0) { clickTime = new Date().getTime() } else { if((new Date().getTime() - clickTime) < 800) { createHeart(e) clickTime = 0 } else { clickTime = new Date().getTime() } } }) const createHeart = (e) => { const heart = document.createElement('i') heart.classList.add('fas') heart.classList.add('fa-heart') const x = e.clientX const y = e.clientY const leftOffset = e.target.offsetLeft const topOffset = e.target.offsetTop const xInside = x - leftOffset const yInside = y - topOffset heart.style.top = `${yInside}px` heart.style.left = `${xInside}px` likeMe.appendChild(heart) times.innerHTML = ++timesClicked setTimeout(() => heart.remove(), 1000) }
Although the code is provided in this tutorial. You can click the button below to get the full folder source code of this project.