Introduction
Python turtle race is a fun project made using turtle graphics library. This is a turtle race that starts from one edge and ends in another edge.
Turtle is an inbuilt module in python that is mostly useful for creating graphics. In this project, we are creating a turtle race. The first turtle to touch the ending area will win. There are six different colors of turtles. This project is easy to use and understand.
First, you must execute the project and enter a color name from your turtle and click enter, the game will start automatically. The program prints whether your turtle win or lose in the console. This is an intermediate-level fun project. We must set up the screen first then give a background color that you like for the screen.
Make a text input that prompts at the beginning and asks the user some information. We must append six turtles with various colors. We further must set the turtle position from where it starts and ends. The source code is along with this article. See the source code and output below how the project looks like.
from turtle import Turtle, Screen import random is_race_on = False screen = Screen() screen.setup(width=500, height=400) screen.bgcolor("black") user_bet = screen.textinput(title="Make your bet", prompt="Which turtle will win the race? Enter a color: ") colors = ["red", "orange", "yellow", "green", "blue", "purple"] y_positions = [-70, -40, -10, 20, 50, 80] all_turtles = [] for turtle_index in range(0, 6): new_turtle = Turtle(shape="turtle") new_turtle.penup() new_turtle.color(colors[turtle_index]) new_turtle.goto(x=-230, y=y_positions[turtle_index]) all_turtles.append(new_turtle) if user_bet: is_race_on = True while is_race_on: for turtle in all_turtles: if turtle.xcor() > 230: is_race_on = False winning_color = turtle.pencolor() if winning_color == user_bet: print(f"You've won! The {winning_color} turtle is the winner!") else: print(f"You've lost! The {winning_color} turtle is the winner!") rand_distance = random.randint(0, 10) turtle.forward(rand_distance) screen.exitonclick()
output
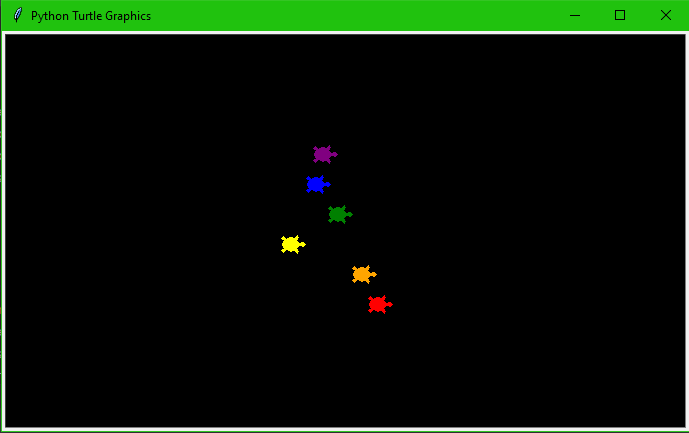