Introduction
Tkinter menu bar is a place where menu items are placed for performing a special tasks. Such as add, new, save, print, etc. It is a very useful bar that is mostly used.
In this Tkinter menu tutorial, we will be creating a menu bar using python GUI library Tkinter. Unlike turtle, Tkinter is a powerful library with many packages used for making GUI applications. There are three types of menu we can create. Top-level, pull down and popup menus. In this project, when you click the menu item you will see a drop down and clicking an item will open a new window. We must import tkinter library.
Syntax for tkinter menu
w = Menu (master, option, ... )
Parameters
- master – It is the parent window for embedding the widget.
- options – It is a list of most commonly used options. It is used for adding functionality.
Some methods used in menu are, add_command (options), add_radiobutton (options), add_checkbox (options), add_separator (), add (type, options),…etc.
The source code for the menu is:
from tkinter import * def donothing(): filewin = Toplevel(root) button = Button(filewin, text = "It works") button.pack() root = Tk() menubar = Menu(root) filemenu = Menu(menubar, tearoff = 1) filemenu.add_command(label = "New", command = donothing) filemenu.add_command(label = "Open", command = donothing) filemenu.add_command(label = "Save", command = donothing) filemenu.add_command(label = "Save as", command = donothing) filemenu.add_command(label = "Close", command = donothing) filemenu.add_separator() filemenu.add_command(label = "Exit", command = root.quit) menubar.add_cascade(label = "File", menu = filemenu) editmenu = Menu(menubar, tearoff = 1) editmenu.add_command(label = "Undo", command = donothing) editmenu.add_separator() editmenu.add_command(label = "Cut", command = donothing) editmenu.add_command(label = "Copy", command = donothing) editmenu.add_command(label = "Paste", command = donothing) editmenu.add_command(label = "Delete", command = donothing) editmenu.add_command(label = "Select All", command = donothing) menubar.add_cascade(label = "Edit", menu = editmenu) helpmenu = Menu(menubar, tearoff = 0) helpmenu.add_command(label = "Help Index", command = donothing) helpmenu.add_command(label = "About,,", command = donothing) menubar.add_cascade(label = "Help", menu = helpmenu) root.config(menu = menubar) root.mainloop()
output
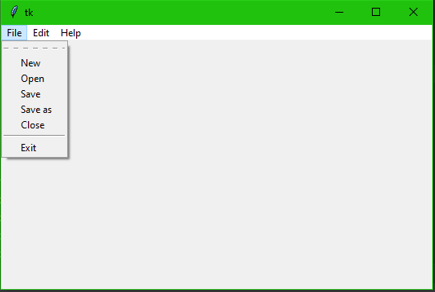