Random shape program is a python turtle library-based project. The program automatically creates random shape on the screen.
In this tutorial, we will be creating a random shape generator using python turtle graphics. Unlike tkinter, Turtle is a powerful library for making GUI applications. The program generates shapes from triangle to decagon. It starts from triangle and stops at the decagon automatically. The program is simple and easy to understand. We must import random function and turtle library for this program. All the shapes build is of different colors. Check the source code and output for random shape project below.
source code
import turtle as t import random tim = t.Turtle() colors = ["red", "blue", "green", "Darkorchid", "wheat", "SlateGray", "yellow"] def draw_shape(num_sides): angle = 360 / num_sides for _ in range(num_sides): tim.forward(100) tim.right(angle) for shape_side_n in range(3, 11): tim.color(random.choice(colors)) draw_shape(shape_side_n)
output
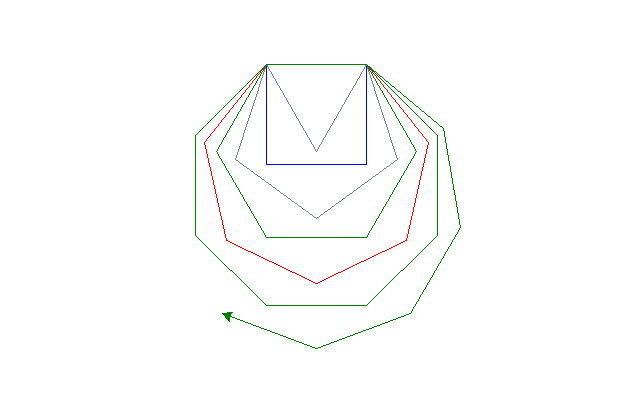