Introduction
What is a canvas? A canvas is a rectangular area for creating drawings and other complex layouts. You can create geometric shapes or insert images.
Canvas is a very useful Tkinter python library. You can often use canvas for creating graphical items. Tkinter is a powerful library with many packages used for making GUI applications. You can draw several widgets in the canvas such as arc bitmap, images, lines, rectangles, text, ovals, polygons, and rectangles. Below there are some examples of widgets created using canvas.
Syntax for canvas
w = Canvas ( Master, option=value, ... )
master – It is the parent window for embedding the widget.
options – It is various parameter used for styling the used widget.
Some common options are: bd (border), bg (background color), height, highlightcolor, relief, width, scrollregion (w, n, e, s : that define over how large an area the canvas can be scrolled , where w = left, n = top, e = right & s = bottom ), xscrollincrement (used for to increase X [multiple of that distance] value of scrolling when scroll bar button is clicked), xscrollcommand (.set() method of the X scrollbar), yscrollincrement, yscrollcommand
You can create the following widget using canvas.
ARC
import tkinter main = tkinter.Tk() canvas = tkinter.Canvas(main, bg = "blue", height = 300, width = 300) chord = 10, 50, 240, 210 #X1,X2,Y1,Y2 canvas.create_arc(chord, start = 0, extent = 90, fill = "red") canvas.pack() main.mainloop()
output:
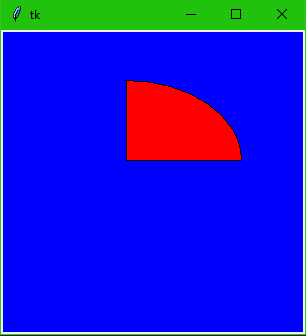
Line
import tkinter main = tkinter.Tk() canvas = tkinter.Canvas(main, bg = "blue", height = 300, width = 300) canvas.create_line(0,0,400,400, fill = "red") canvas.pack() main.mainloop()
output:
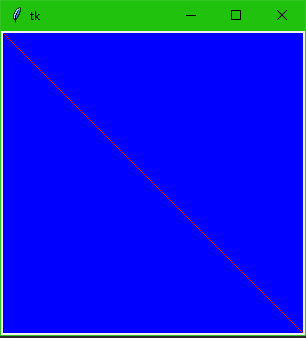
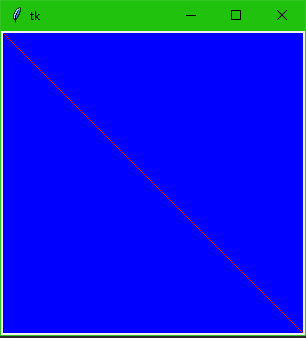
Ploygon
import tkinter main = tkinter.Tk() canvas = tkinter.Canvas(main, bg = "blue", height = 300, width = 300) canvas.create_polygon(55, 85, 155, 85, 105, 180, 55, 85, fill = "yellow") canvas.pack() main.mainloop()
output:
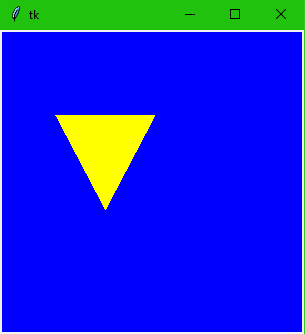
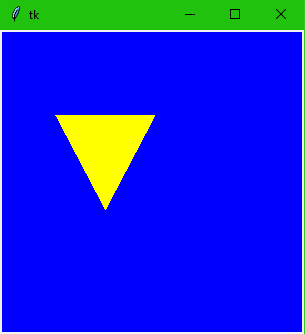
Image
import tkinter main = tkinter.Tk() canvas = tkinter.Canvas(main, bg = "blue", height = 300, width = 300) image (photoimage takes .gif or pgm/ppm) image = tkinter.PhotoImage(file = "sponge.gif") canvas.create_image(image.width()/2, image.width() / 2, anchor = tkinter.CENTER, image = image) canvas.pack() main.mainloop()
The image widget displays image on the canvas.