Introduction
Exception handling is the process of handling errors when the program runs. It is the process of responding to the occurrence of the exceptions.
An exception is a python object that represents error. Exception are raised when the program encounters an error during its execution. The keyword that are used in exception handling is try, except, else, and finally. The try: block contains one or more statements which are likely to encounter an exception.
If the statements in this block are executed without an exception, then it is skipped. If there is some exception, the program flow is transferred to the except block. The statements in the except block are meant to handle the cause of the exception appropriately. Like, returning an appropriate error message.
try: #statements in try block except: #executed when error in try block else: #executed if try block is error-free finally: #executed irrespective of exception occurred or not
While the except block is executed if the exception occurs inside the try block, the else block gets processed if the try block is found to be exception free. The finally block consists of statements that should be processed if there is an exception occurring in the try block or not. The finally block executes rest of the code.
Example for exception handling
try: file = open("a_file.txt") a_dictionary = {"key": "value"} print(a_dictionary["key"]) except FileNotFoundError: file = open("a_file.txt", "w") file.write("Something") except KeyError as error_message: print(f"The key {error_message} does not exist.") else: content = file.read() print(content) finally: raise KeyError("This is the error i made while exception handling")
output
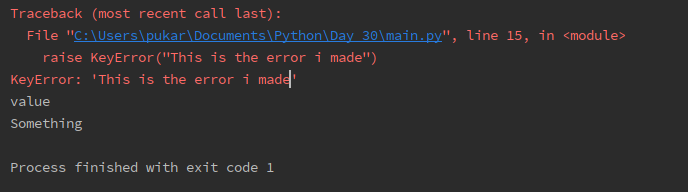