Introduction
Unit converter program is a python Tkinter based project. It generally converts one type of unit to another unit. Such as m to km, cm to m, …etc.
In this tutorial, we will be creating miles to kilometer unit converter. Unlike turtle, Tkinter is a powerful library for making GUI applications. This is a simple program made using the Tkinter library. The program asks the user to enter the miles. You must click the calculate button to see the converted units in kilometres.
Similarly, you can add more units in this project. We must import tkinter library. We are using python tkinter widgets like labels, entries, buttons, etc. A function is created for calculating miles into km. When the button is pressed the answer is displayed. See the code and output below.
Source code
from tkinter import * def miles_to_km(): miles = float(miles_input.get()) km = miles * 1.609 km_result_label.config(text=f"{km}") window = Tk() window.title("Miles to Km convertor") window.config(padx=20, pady=20) miles_input = Entry(width=7) miles_input.grid(column=1, row=0) miles_label = Label(text="Miles") miles_label.grid(column=2, row=0) is_equal_label = Label(text="is equal to") is_equal_label.grid(column=0, row=1) km_result_label = Label(text="0") km_result_label.grid(column=1, row=1) km_label = Label(text="Km") km_label.grid(column=2, row=1) calc_button = Button(text="Calculate", command=miles_to_km) calc_button.grid(column=1, row=2) window.mainloop()
Output
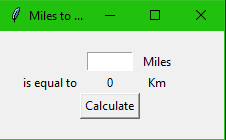
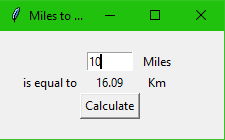