Introduction
Sound board project is an audio-based web project. It is coded in HTML5, CSS, and JavaScript. This project deals with playing sound on button click.
In this tutorial, we will be creating a static web page with sound. First, we need to create a folder sound board with html, css, sound, and js files. We are using a container which includes all the audio parts. We will create a couple of simple button that plays sound. We will use audio html tag to insert sound. Buttons are styled with CSS.
The functionality of clicking button is added via Javascript code. All the styling is done using CSS. Javascript makes it functional, when you press button the sound starts to play and when you press another the previous sound stops and another sound plays. See the screenshot below.
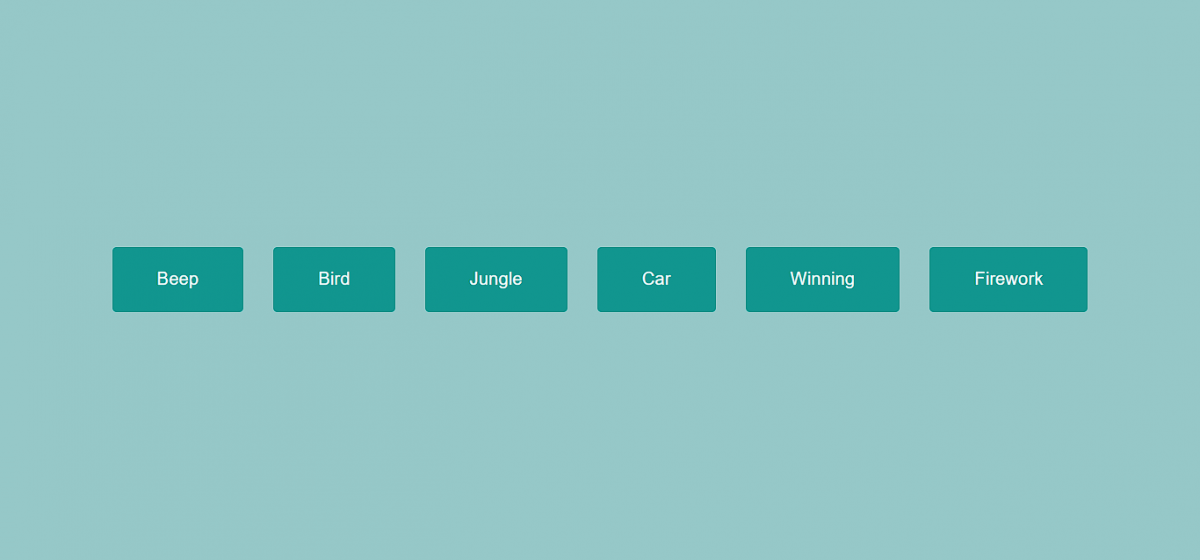
index.html
HTML will be used for displaying a buttons and text on screen. Download sounds from free sound library or you can use your own sound from your device.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/5.15.2/css/all.min.css" integrity="sha512-HK5fgLBL+xu6dm/Ii3z4xhlSUyZgTT9tuc/hSrtw6uzJOvgRr2a9jyxxT1ely+B+xFAmJKVSTbpM/CuL7qxO8w==" crossorigin="anonymous" /> <link rel="stylesheet" href="style.css"> <title>Sound board</title> </head> <body> <audio id = "Beep" src="sounds/beep.mp3"></audio> <audio id = "Bird" src="sounds/bird.mp3"></audio> <audio id = "Jungle" src="sounds/jungle.mp3"></audio> <audio id = "Car" src="sounds/car.mp3"></audio> <audio id = "Winning" src="sounds/winning.mp3"></audio> <audio id = "Firework" src="sounds/firework.mp3"></audio> <div id="buttons"></div> <script src="script.js"></script> </body> </html>
Sound board CSS
style.css
We will be using CSS for styling the displayed buttons and text on sound board project.
@import url('https://fonts.googleapis.com/css?Muli&display=swap'); * { box-sizing: border-box; } body { background-color: rgb(150, 200, 200); font-family: 'Muli', sans-serif; display: flex; flex-wrap: wrap; align-items: center; justify-content: center; text-align: center; height: 100vh; overflow: hidden; margin: 0; } .btn { background-color: rgb(17, 150, 143); border-radius: 5px; border: none; color: #fff; margin: 1rem; padding: 1.5rem 3rem; font-size: 1.2rem; font-family: inherit; cursor: pointer; } .btn:hover { opacity: 0.9; } .btn:focus { outline: none; }
The Javascript code is added for making button clickable. A even listener is added on each button that plays sound on click. This project is responsive from small to large device. Check the js code below.
script.js
const sounds = ['Beep', 'Bird', 'Jungle', 'Car', 'Winning', 'Firework'] sounds.forEach(sound => { const btn = document.createElement('button') btn.classList.add('btn') btn.innerText = sound btn.addEventListener('click', () => { stopSong() document.getElementById(sound).play() }) document.getElementById('buttons').appendChild(btn) }) function stopSong() { sounds.forEach(sound => { const song = document.getElementById(sound) song.pause() song.currentTime = 0; }) }
Although the code is provided in this tutorial. You can click the button below to get the source code of this project.
I am sure this paragraph has touched all the internet users,
its really really pleasant post on building up new blog.