Introduction
Rotating nav is a web based project. This tutorial deals with creating a rotating navigation bar. It is coded in HTML5, CSS, and JavaScript.
In this tutorial we will be creating a rotating navigation. First we need to create a folder with images folder and files. We will be using images, icons and some text. We will create a simple webpage which shows the rotating nav animation. We will use javascript for rotating webpage on button click. It is a static webpage which does not include any functionality.
Further we use font awesome icons. CSS will be used for animating and styling the rotating nav project. There is a top left navigation button which rotate the webpage on click. It’s all about playing with css and js. We create a container and all the stuff will be styled inside container. You can use cdnjs website for CDN of the different libraries. See the screenshot below.
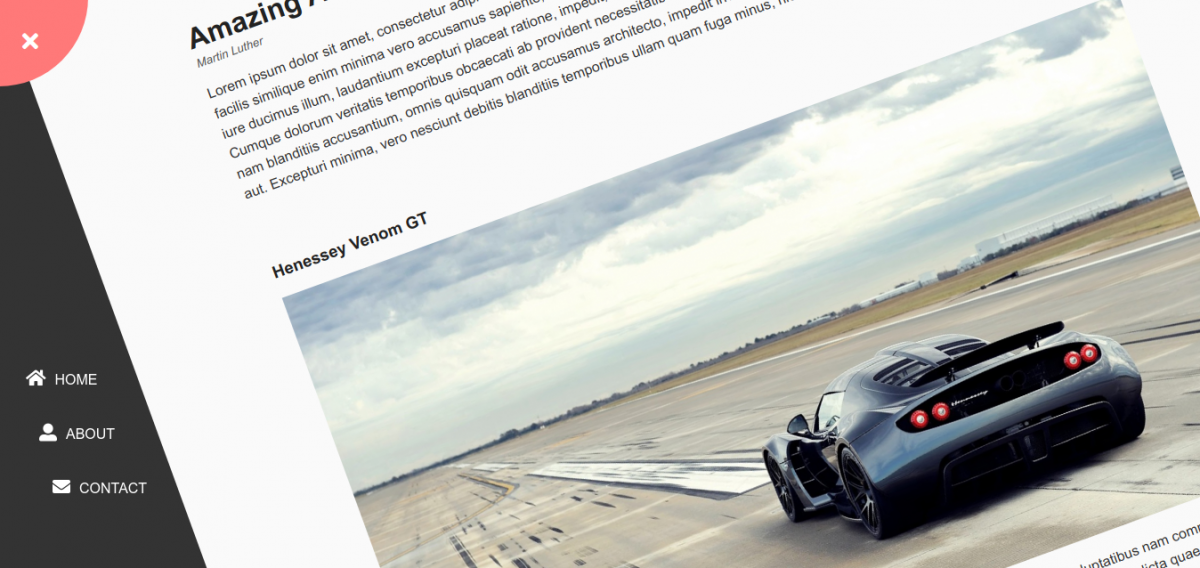
Html will be used for placing the image and text on screen. We are using dummy text for this project. Check the code below.
index.html
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/5.15.2/css/all.min.css" integrity="sha512-HK5fgLBL+xu6dm/Ii3z4xhlSUyZgTT9tuc/hSrtw6uzJOvgRr2a9jyxxT1ely+B+xFAmJKVSTbpM/CuL7qxO8w==" crossorigin="anonymous" /> <link rel="stylesheet" href="style.css"> <title>Rotating Nav</title> </head> <body> <div class="container"> <div class="circle-container"> <div class="circle"> <button id="close"> <i class="fas fa-times"></i> </button> <button id="open"> <i class="fas fa-bars"></i> </button> </div> </div> <div class="content"> <h1>Amazing Article</h1> <small>Martin Luther</small> <p>Lorem ipsum dolor sit amet, consectetur adipisicing elit. Soluta ea, nesciunt, recusandae maiores illo animi odio labore possimus voluptates facilis similique enim minima vero accusamus sapiente, accusantium sequi totam. Nemo, aliquid hic? Deserunt nobis voluptatum corporis iure ducimus illum, laudantium excepturi placeat ratione, impedit, illo laboriosam eaque harum sequi officiis ullam repudiandae voluptates. Cumque dolorum veritatis temporibus obcaecati ab provident necessitatibus recusandae molestiae sint nostrum esse fugiat debitis atque nam blanditiis accusantium, omnis quisquam odit accusamus architecto, impedit inventore maiores excepturi! Quis impedit doloribus iure ex aut. Excepturi minima, vero nesciunt debitis blanditiis temporibus ullam quam fuga minus, hic consectetur!</p> <br></br> <h3>Henessey Venom GT</h3> <img src="images/3.jpg" alt=""> <p>Lorem ipsum dolor sit amet consectetur adipisicing elit. Quam a quaerat excepturi mollitia culpa, nulla voluptatibus nam commodi tenetur eaque magni, eos nobis unde sit! Placeat doloremque sed officiis, suscipit porro tempora ab ipsum possimus vel dicta quae earum, reiciendis aut laborum! Voluptatum distinctio nostrum laboriosam. Dolorem error rem tempore ad maxime quae temporibus laboriosam, veniam dignissimos, minus quasi perspiciatis, illum sit ducimus quos quod at officia earum ipsam fugiat eveniet? Maxime omnis iusto ipsa.</p> </div> </div> <nav> <ul> <li><i class="fas fa-home"></i>Home</li> <li><i class="fas fa-user-alt"></i>About</li> <li><i class="fas fa-envelope"></i>Contact</li> </ul> </nav> <script src="script.js"></script> </body> </html>
Rotating nav css code
We will be using CSS for styling the image, content and navigation button. Check the css code how the button is styled and transition is added.
Style.css
@import url('https://fonts.googleapis.com/css?Muli&display=swap'); * { box-sizing: border-box; } body { font-family: 'Muli', sans-serif; background-color: #333; color: #222; overflow-x: hidden; margin: 0; } .container { background-color: #fafafa; transform-origin: top left; transition: transform 0.5s linear; width: 100vw; min-height: 100vh; padding: 50px; } .container.show-nav { transform: rotate(-20deg); } .circle-container { position: fixed; top: -100px; left: -100px; } .circle { background-color: #ff7979; height: 200px; width: 200px; border-radius: 50%; position: relative; transition: transform 0.5s linear; } .container.show-nav .circle { transform: rotate(-70deg); } .circle button { cursor: pointer; position: absolute; top: 50%; left: 50%; height: 100px; background: transparent; border: 0; font-size: 26px; color: #fff; } .circle button:focus { outline: none; } .circle button#open { left: 60%; } .circle button#close { top: 60%; transform: rotate(90deg); transform-origin: top left; } .container.show-nav + nav li { transform: translateX(0); transition-delay: 0.3s; } nav { position: fixed; bottom: 40px; left: 0; z-index: 100; } nav ul { list-style-type: none; padding-left: 30px; } nav ul li { text-transform: uppercase; color: #fff; margin: 40px 0; /* transform: translateX(-100%); */ transition: transform 0.4s ease in; } nav ul li i { font-size: 20px; margin-right: 10px; } nav ul li + li { margin-left: 15px; transform: translateX(-150%); } nav ul li + li +li { margin-left: 30px; transform: translateX(-200%); } .content img { max-width: 100%; } .content { max-width: 1000px; margin: 50px auto; } .content h1 { margin: 0; } .content small { color: #555; font-style: italic; } .content p { color: #333; line-height: 1.5; }
Rotating nav js code
The javascript will be used for adding click event listener on the nav icon. This project is responsive from small to large device. Click the bar nav icon to rotate and show the menu items. If you click the close icon the page regains the default position. Check the js code below
script.js
const open = document.getElementById('open') const close = document.getElementById('close') const container = document.querySelector('.container') open.addEventListener('click', () => container.classList.add('show-nav')) close.addEventListener('click', () => container.classList.remove('show-nav'))
Although the code is provided in this tutorial. You can click the button below to get the source code of this project.