Introduction
Password or Passcode generator is a process of generating a random passcode that is very hard to guess. This project is using python programming.
In this tutorial, we will be creating a passcode generator project using python programming. While executing the project, it will ask us to enter the length of Passcode, length of symbols, length of numbers, etc. After you provide the information the Passcode will be generated that is hard to guess. This project has a command-line interface. Well, Passcode is one of the most sensitive pieces of information in the computer world.
The strongest the Passcode is, the secure you will be. Today everything requires a Passcode. If you have a weak Passcode and is easily cracked the sensitive information on your device will be stolen by someone else. There are various types of Passcode checker websites where you can check how strong is your Passcode and the time require to crack your Passcode. Some websites are Password meter, online domain tools, my1login.com, etc, and many more.
We are creating this Passcode generator writing a python code. The python code is divided into two steps.
Step-1 Importing, creating lists, user input
letters = ['a', 'b', 'c', 'd', 'e', 'f', 'g', 'h', 'i', 'j', 'k', 'l', 'm', 'n', 'o', 'p', 'q', 'r', 's', 't', 'u', 'v', 'w', 'x', 'y', 'z', 'A', 'B', 'C', 'D', 'E', 'F', 'G', 'H', 'I', 'J', 'K', 'L', 'M', 'N', 'O', 'P', 'Q', 'R', 'S', 'T', 'U', 'V', 'W', 'X', 'Y', 'Z'] numbers = ['0', '1', '2', '3', '4', '5', '6', '7', '8', '9'] symbols = ['!', '#', '$', '%', '&', '(', ')', '*', '+'] print(""" |++++++++++++| |--passcode--| |++++++++++++| | | | | _| |________| |_ .' |_| |_| '. '._____ ____ _____.' | .'____'. | '.__.'.' '.'.__.' '.__ | 0000 | __.' | '.'.____.'.' | '.____'.____.'____.'LGB '.________________.' """) print("Welcome to the Passcode Generator!") nr_letters= int(input("How many letters would you like in your Passcode?\n")) nr_symbols = int(input(f"How many symbols would you like?\n")) nr_numbers = int(input(f"How many numbers would you like?\n"))
First we import random function. After that, we create some lists, letters with uppercase and lowercase, numbers, and symbols. While generating a Passcode, the code will use random letters or symbols or numbers to display a new Passcode. We added some user input function and assigned to the variable that ask the user to input asked information.
Step 2 – Making the code functions properly
Passcode_list = [] // passcode generator for char in range(1, nr_letters+1): Passcode_list.append(random.choice(letters)) for char in range(1, nr_symbols+1): Passcode_list += random.choice(symbols) for char in range(1, nr_numbers + 1): Passcode_list += random.choice(numbers) print(Passcode_list) random.shuffle(Passcode_list) print(Passcode_list) Passcode = "" for char in Passcode_list: Passcode += char print(f"Passcode {Passcode}")
We create an empty list to store a Passcode. We use for loop in this project. The for loop will initialize the number entered by user between the range 1 and user input. The random choice will generate the random letters, symbols, numbers, and append to the empty Passcode list.
If we print the Passcode, we will get the number in sequence. Such as letters, numbers, and symbols. E.g. qWeqAO123$%£ etc. We need to shuffle them. We use random.shuffle function for shuffling our Passcode. E.g. We3e4e*er%^5&6£Dr etc. Now we must print the Passcode from the Passcode list. Check the screenshot of the output below.
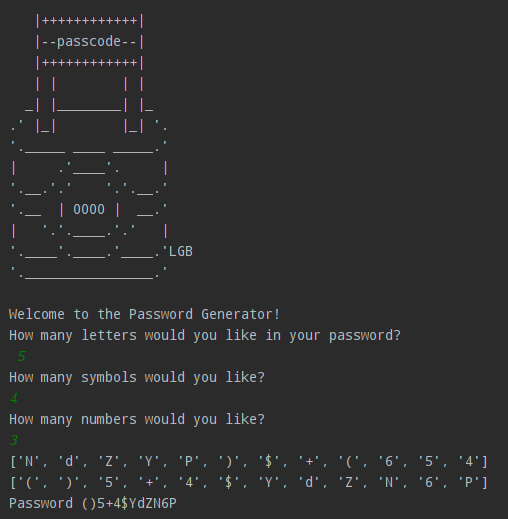
How to use this passcode generator project?
- Copy the code to your editor.
- Execute the project.
- Use the Passcode.