Introduction
Movie app is a movie-based web project. It is coded in HTML5, CSS, and JavaScript. It shows information about the different movies released.
In this tutorial movie app, we will be creating a simple movie app. First, we need to create a folder with HTML, CSS, and js files. This project’s functionality is made with Javascript. The movie app project has a simple layout with grid boxes. There is a search box in the top right corner. When the movie box-shadow has hovered you will see the movie information.
The movie information displays with animation from bottom to top. The project shows movie ratings with different colors and numbers. We will be using a movie API which will give access to our movie app project. You need to run the index.html file in the browser to run the project. See the screenshot below.
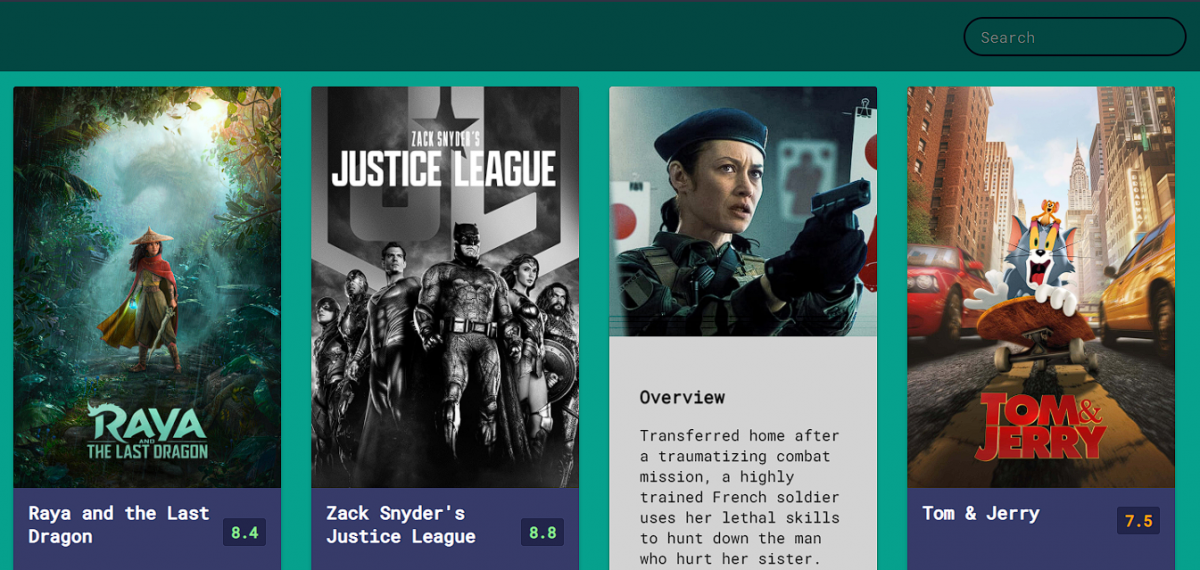
Movie app project source code
HTML
HTML will be used for displaying movies on screen. First, we create HTML code as a placeholder and then moved it to a JavaScript file. You will see a few HTML codes here. We are using the movie database API to display movies in our project.
index.html
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/5.15.2/css/all.min.css" integrity="sha512-HK5fgLBL+xu6dm/Ii3z4xhlSUyZgTT9tuc/hSrtw6uzJOvgRr2a9jyxxT1ely+B+xFAmJKVSTbpM/CuL7qxO8w==" crossorigin="anonymous" /> <link rel="stylesheet" href="style.css"> <title>Movie</title> </head> <body> <header> <form id="form"> <input type="text" id="search" class="search" placeholder="Search"> </form> </header> <main id="main"> </main> <script src="script.js"></script> </body> </html>
CSS
We will be using CSS for styling the whole project. Such as background color, icons, text, aligning, flex-items, colors and many more.
style.css
@import url('https://fonts.googleapis.com/css?family=Roboto+Mono&display=swap'); * { box-sizing: border-box; } body { background-color: #06a18d; font-family: 'Roboto Mono', sans-serif; margin: 0; } header { padding: 1rem; display: flex; justify-content: flex-end; background-color: #034742; } .search { background-color: transparent; border: 2px solid #04040e; border-radius: 50px; font-family: inherit; font-size: 1rem; padding: .5rem 1rem; color: #fff; } .search:placeholder { color: #e6e6ec; } .search:focus { outline: none; background-color: rgb(7, 141, 141); } main { display: flex; flex-wrap: wrap; } .movie { width: 300px; margin: 1rem; background-color: #373b69; box-shadow: 0 4px 5px rgba(0, 0, 0, 0.2); position: relative; overflow: hidden; border-radius: 3px; } .movie img { width: 100%; } .movie-info { color: #fff; display: flex; align-items: center; justify-content: space-between; padding: 0.5rem 1rem 1rem; letter-spacing: 0.5ox; } .movie-info h3 { margin-top: 0; } .movie-info span { background-color: #22254b; padding: .25rem 0.5rem; border-radius: 3px; font-weight: bold; } .movie-info span.green { color: lightgreen; } .movie-info span.orange { color: orange; } .movie-info span.red { color: red; } .overview { background-color: lightgrey; padding: 2rem; position: absolute; left: 0; bottom: 0; right: 0; max-height: 100%; transform: translateY(101%); transition: transform 0.3s ease-in; } .movie:hover .overview { transform: translateY(0); }
JS
The JavaScript code is used for fetching the movie from the API. Also it is used for making search functionality. The JavaScript works as backend in this project. Check the code below.
scripts.js
const API_URL = 'https://api.themoviedb.org/3/discover/movie?sort_by=popularity.desc&api_key=23381f9d606c6cff00c6543b3446c4ad&page=1' const IMG_PATH= 'https://image.tmdb.org/t/p/w1280' const SEARCH_API = 'https://api.themoviedb.org/3/search/movie?api_key=23381f9d606c6cff00c6543b3446c4ad&query=" ' const main = document.getElementById('main') const form = document.getElementById('form') const search = document.getElementById('search') getMovies(API_URL) async function getMovies(url) { const res = await fetch(url) const data = await res.json() showMovies(data.results) } function showMovies(movies) { MediaDeviceInfo.innerHTML = '' movies.forEach(movie => { const {title, poster_path, vote_average, overview} = movie const movieEl = document.createElement('div') movieEl.classList.add('movie') movieEl.innerHTML = ` <img src="${IMG_PATH + poster_path}" alt="${title}"> <div class="movie-info"> <h3>${title}</h3> <span class="${getClassByRate(vote_average)}">${vote_average}</span> </div> <div class="overview"> <h3>Overview</h3> ${overview} </div> ` main.appendChild(movieEl) }); } function getClassByRate(vote) { if(vote >= 8) { return 'green' } else if(vote >=5) { return 'orange' } else { return 'red' } } form.addEventListener('submit', (e) => { e.preventDefault() const searchTerm = search.value if(searchTerm && searchTerm !== '') { getMovies(SEARCH_API + searchTerm) search.value = '' } else { window.location.reload() } })
Although the code is provided in this tutorial. You can click the button below to get the full source code of this project.