Introduction
Just for laugh challenge is a joke-based web project. It is coded in HTML5, CSS, and JavaScript. This project deals with displaying a joke from the server.
In this tutorial, we will be creating a static web page that displays jokes. First, we need to create a just for laugh folder with html, css, and js files. We are using a container which includes text and button. We are creating essential parts using CSS. We will be fetching a joke from the server using JavaScript.
The project has a simple layout with background color and box layout in the middle that displays a joke. A next button will show a new joke on every click. The fetching data and clicking button functionality is added via JavaScript code. You need to run index.html file in the browser to run the project. See the screenshot below.
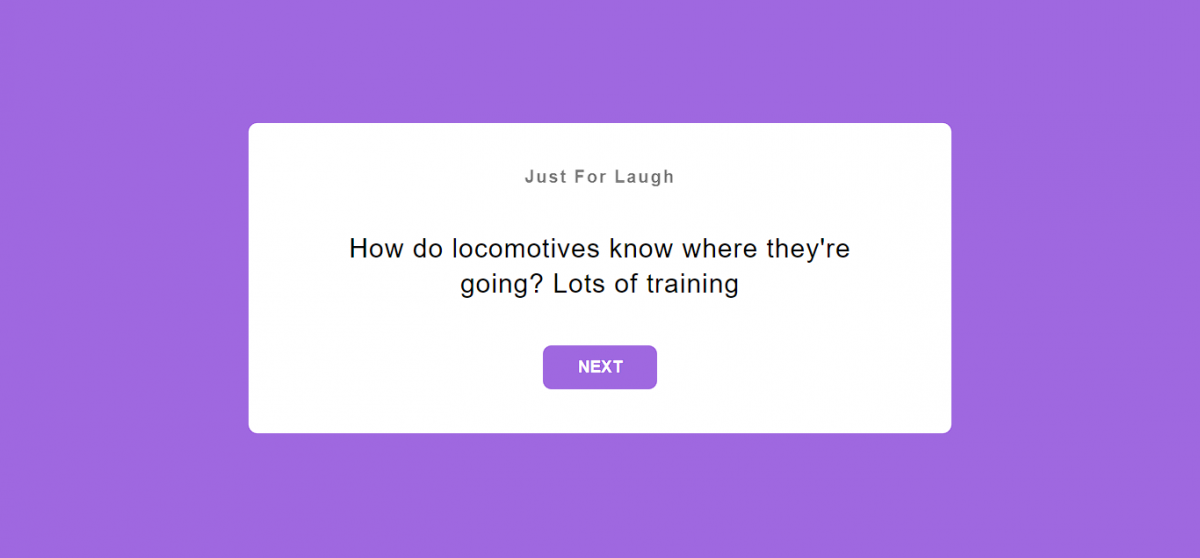
Just for laugh source code
HTML will be used for displaying a buttons and text on the screen. You can check this website icanhazdadjoke.com for the jokes.
index.html
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/5.15.2/css/all.min.css" integrity="sha512-HK5fgLBL+xu6dm/Ii3z4xhlSUyZgTT9tuc/hSrtw6uzJOvgRr2a9jyxxT1ely+B+xFAmJKVSTbpM/CuL7qxO8w==" crossorigin="anonymous" /> <link rel="stylesheet" href="style.css"> <title>Just for laugh</title> </head> <body> <div class="container"> <h3>Just For Laugh</h3> <div id="joke" class="joke"> </div> <button id="jokeBtn" class="btn">NEXT</button> </div> <script src="script.js"></script> </body> </html>
We will be using CSS for styling the whole project. Such as background colour, box, text, buttons, colours and many more.
style.css
@import url('https://fonts.googleapis.com/css?Muli&display=swap'); * { box-sizing: border-box; } body { background-color: #9f68e0; font-family: 'Muli', sans-serif; display: flex; flex-direction: column; align-items: center; justify-content: center; height: 100vh; overflow: hidden; margin: 0; padding: 20px; } .container { background-color: #fff; border-radius: 10px; box-shadow: 0 10px 20px rgba(0, 0, 0, 0.1), 0 6ox 6px rgba(0, 0, 0, 0.1); padding: 50px 20px; text-align: center; max-width: 100%; width: 800px; } h3 { margin: 0; opacity: 0.5; letter-spacing: 2px; } .joke { font-size: 30px; letter-spacing: 1px; line-height: 40px; margin: 50px auto; max-width: 600px; } .btn { background-color: #9f68e0; color: #fff; border: 0; border-radius: 10px; box-shadow: 0 10px 20px rgba(0, 0, 0, 0.1), 0 6ox 6px rgba(0, 0, 0, 0.1); padding: 14px 40px; font-size: 19px; cursor: pointer; font-weight: 600; } .btn:active { transform: scale(0.99); } .btn:focus { outline: 0; }
The JavaScript code is added for making the button clickable and etching the jokes from the server. An event listener is added on button that shows new jokes on click. This project is responsive from small to large devices. Check the js code below.
script.js
const jokeEl = document.getElementById('joke') const jokeBtn = document.getElementById('jokeBtn') jokeBtn.addEventListener('click', generateJoke) generateJoke() function generateJoke() { const config = { headers: { 'Accept': 'application/json' } } fetch('https://icanhazdadjoke.com', config) .then((res) => res.json()) .then((data) => { jokeEl.innerHTML = data.joke }) }
Although the code is provided in this tutorial. You can click the button below to get the full source code of this project.