Introduction
Blur loading is a web-based project. It is coded in HTML5, CSS, and JavaScript. This tutorial deals with creating a blur loading image with the counter.
In this tutorial, we will be creating a blur loading page. First, we need to create a folder with images folder and files. We will be using images and numbers. We will create a simple webpage that shows the blurry loading effect. We will use CSS and JavaScript for making blur loading.
It is a static webpage that does not include any functionality. The animation starts from blur with counting number from 0 to 100 and we can see clear image. Every time we refresh the page the animation starts. It’s all about playing with CSS and js. See the screenshot below.
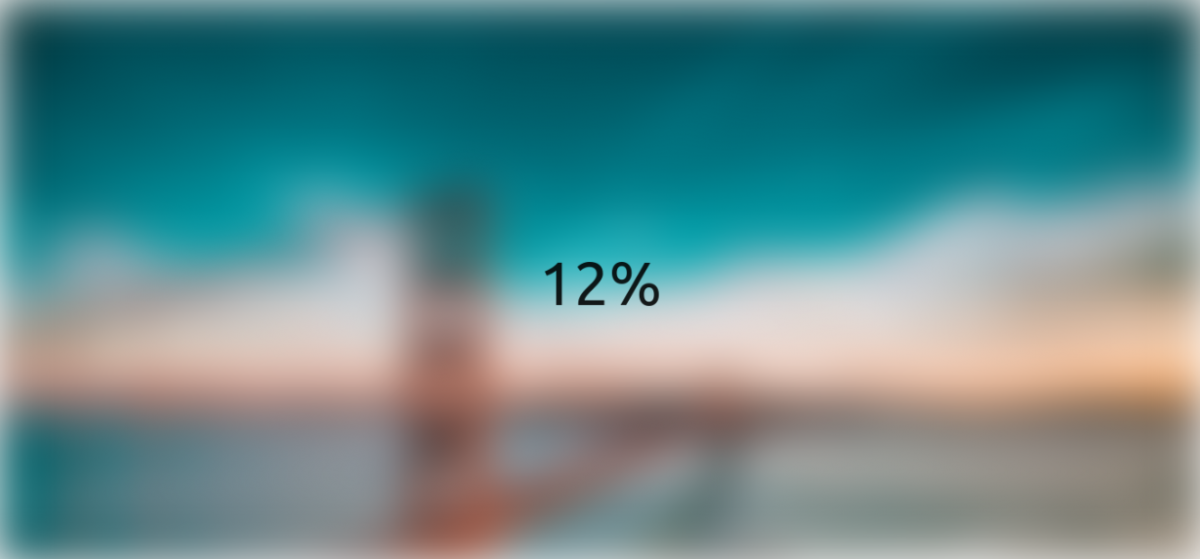
index.html
Html will be used for placing the image and text on screen. We are using free image from unspalsh website.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/ font-awesome/5.15.2/css/all.min.css" integrity="sha512-HK5fgLBL+xu6dm /Ii3z4xhlSUyZgTT9tuc/hSrtw6uzJOvgRr2a9jyxxT1ely+B+xFAmJKVSTbpM/CuL7qx O8w==" crossorigin="anonymous" /> <link rel="stylesheet" href="style.css"> <title>Blur</title> </head> <body> <section class="bg"></section> <div class="loading-text">0%</div> <script src="script.js"></script> </body> </html>
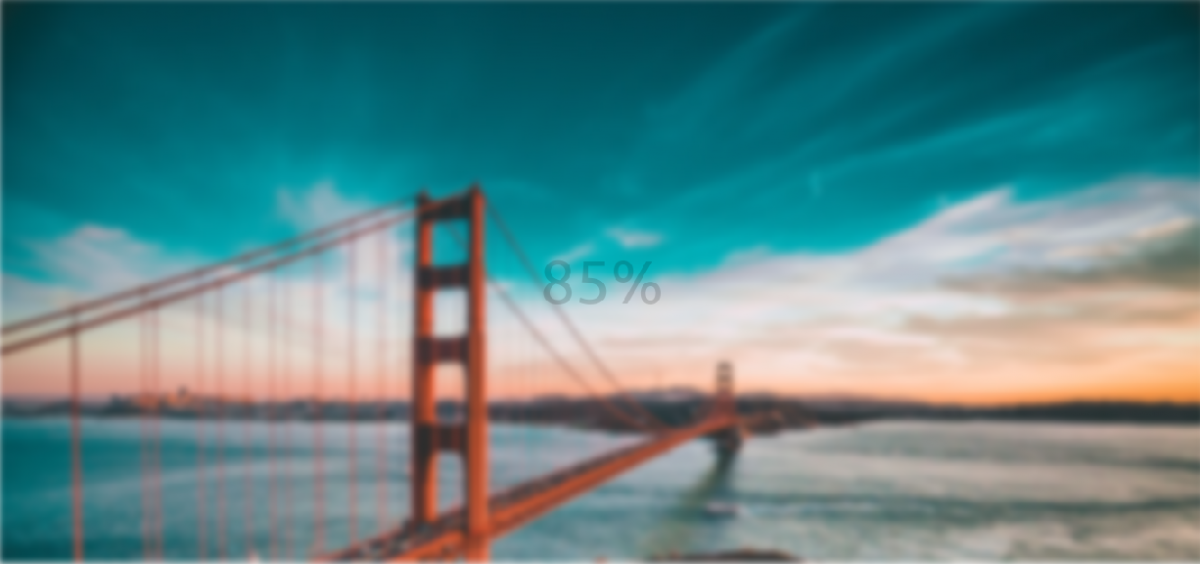
Blur loading project css
We will be using CSS for styling and blurring the image, fixing position, colour and text. See the code below.
style.css
@import url('https://fonts.googleapis.com/css?family=Ubuntu'); * { box-sizing: border-box; } body { font-family: 'Ubuntu', sans-serif; display: flex; align-items: center; justify-content: center; height: 100vh; overflow: hidden; margin: 0; } .bg { background: url(https://images.unsplash.com/photo-1449034446853-66c86144b0ad?ixid=MXwxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHw%3D&ixlib=rb-1.2.1&auto=format&fit=crop&w=1366&q=80); position: absolute; top: -30; left: -30; width: calc(100vw + 60px); height: calc(100vh + 60px); width: 100vw; height: 100vh; z-index: -1; filter: blur(0px); } .loading-text { font-family: 50px; color: #000; font-size: 70px; font-weight: 400; }
Blur loading project js
The javascript will be used for setting the time interval between 0 and 100. When user refreshes the page they must see the blur effect with counting numbers. This project is responsive from small to large devices. Check the js code below.
script.js
const loadText = document.querySelector('.loading-text') const bg = document.querySelector('.bg') let load = 0 let int = setInterval(blurring, 30) function blurring() { load++ if(load > 99) { clearInterval(int) } loadText.innerText = `${load}%` loadText.style.opacity = scale(load, 0, 100, 1, 0) bg.style.filter = `blur(${scale(load, 0, 100, 30, 0)}px)` } const scale = (num, in_min, in_max, out_min, out_max) => { return(num - in_min) * (out_max - out_min) / (in_max - in_min) +out_min; }
Although the code is provided in this tutorial. You can click the button below to get the source code of this project.